728x90
반응형
Java 자주발생하는 오류 정리 #20
Frequent Java Cleanup Errors #20
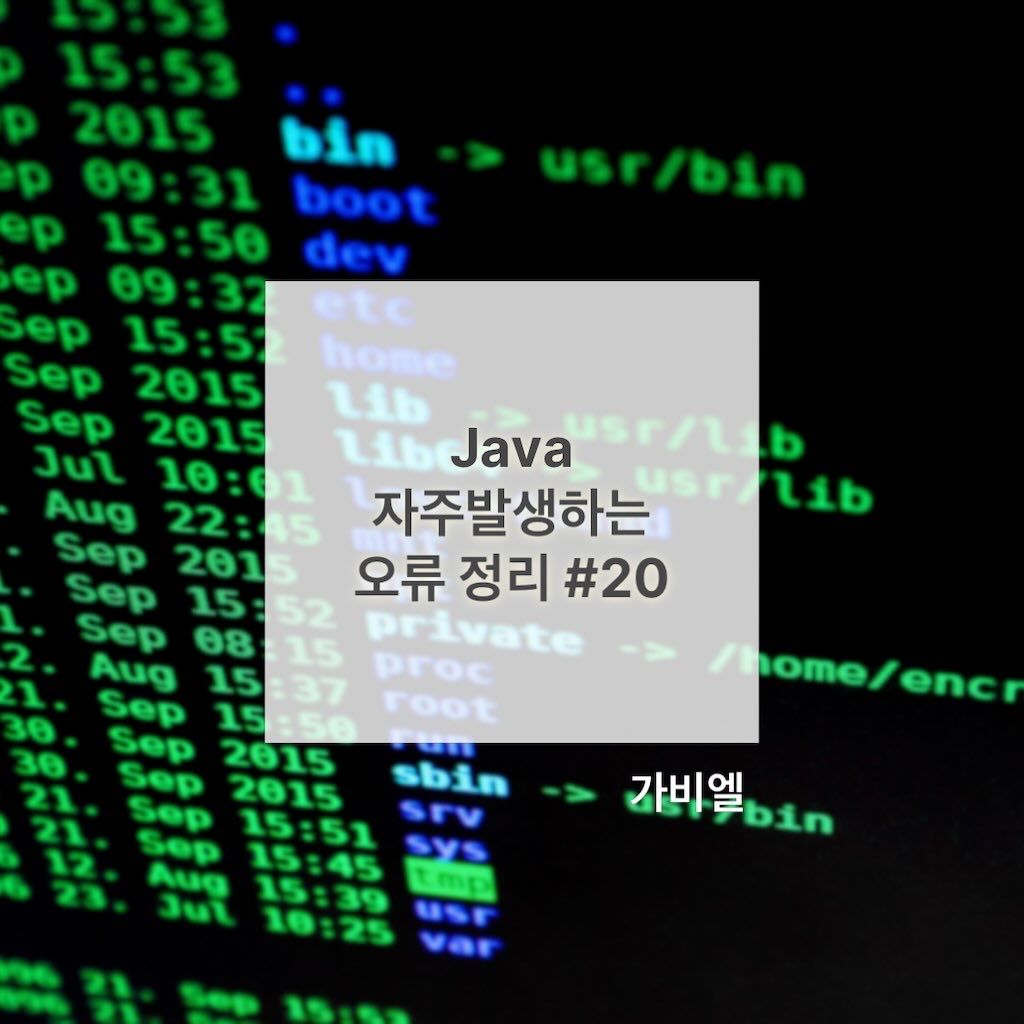
NotActiveException
NotActiveException은 활성화되지 않은 그래프 노드를 역직렬화하려고 할 때 발생하는 예외입니다. 주로 직렬화 및 역직렬화에서 발생합니다.
취약점
- NotActiveException은 주로 직렬화와 관련이 있으며, 보안 취약점을 나타내지는 않습니다. 그러나 역직렬화되는 객체의 활성 상태를 확인하고 객체를 활성화하는 것이 중요합니다.
오류상황
import java.io.*;
public class NotActiveExceptionExample {
public static void main(String[] args) {
try {
FileInputStream fileIn = new FileInputStream("employee.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Employee employee = (Employee) in.readObject(); // 활성화되지 않은 객체를 역직렬화 시도할 때 NotActiveException 발생
in.close();
fileIn.close();
} catch (NotActiveException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
솔루션
import java.io.*;
public class NotActiveExceptionSolution {
public static void main(String[] args) {
try {
FileInputStream fileIn = new FileInputStream("employee.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Employee employee = (Employee) in.readObject();
if (employee.isActive()) {
// 객체 활성화 상태인 경우 처리
} else {
// 객체 비활성화 상태인 경우 처리
}
in.close();
fileIn.close();
} catch (NotActiveException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
솔루션 설명
- 역직렬화되는 객체의 활성 상태를 확인하고, 객체를 활성화하여 NotActiveException을 방지할 수 있습니다.
NotDirectoryException
NotDirectoryException은 디렉토리가 아닌 파일을 디렉토리로 사용하려고 할 때 발생하는 예외입니다. 주로 파일 시스템 작업에서 발생합니다.
취약점
- NotDirectoryException은 주로 파일 시스템 작업과 관련이 있으며, 보안 취약점을 나타내지는 않습니다. 그러나 올바른 디렉토리를 사용하고 파일이 아닌 디렉토리를 확인하는 것이 중요합니다.
오류상황
import java.nio.file.*;
public class NotDirectoryExceptionExample {
public static void main(String[] args) {
try {
Path path = Paths.get("file.txt");
DirectoryStream<Path> stream = Files.newDirectoryStream(path); // 파일을 디렉토리로 사용하려고 할 때 NotDirectoryException 발생
} catch (NotDirectoryException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
}
솔루션
import java.nio.file.*;
public class NotDirectoryExceptionSolution {
public static void main(String[] args) {
try {
Path path = Paths.get("directory");
if (Files.isDirectory(path)) {
DirectoryStream<Path> stream = Files.newDirectoryStream(path); // 올바른 디렉토리 사용
} else {
// 디렉토리가 아닌 파일에 대한 처리
}
} catch (NotDirectoryException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
}
솔루션 설명
- 올바른 디렉토리를 사용하고, 파일이 아닌 디렉토리를 확인하여 NotDirectoryException을 방지할 수 있습니다.
NotSerializableException
NotSerializableException은 직렬화할 수 없는 객체를 직렬화하려고 할 때 발생하는 예외입니다. 주로 직렬화와 관련된 작업에서 발생합니다.
취약점
- NotSerializableException은 주로 직렬화와 관련이 있으며, 보안 취약점을 나타내지는 않습니다. 그러나 직렬화 가능한 객체만 직렬화하고 객체의 직렬화 가능 여부를 확인하는 것이 중요합니다.
오류상황
import java.io.*;
public class NotSerializableExceptionExample {
public static void main(String[] args) {
try {
NonSerializableObject nonSerializableObject = new NonSerializableObject();
FileOutputStream fileOut = new FileOutputStream("object.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(nonSerializableObject); // 직렬화할 수 없는 객체를 직렬화 시도할 때 NotSerializableException 발생
out.close();
fileOut.close();
} catch (NotSerializableException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
class NonSerializableObject {
// 직렬화할 수 없는 객체
}
솔루션
import java.io.*;
public class NotSerializableExceptionSolution {
public static void main(String[] args) {
try {
SerializableObject serializableObject = new SerializableObject();
FileOutputStream fileOut = new FileOutputStream("object.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(serializableObject); // 직렬화 가능한 객체를 직렬화
out.close();
fileOut.close();
} catch (NotSerializableException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
class SerializableObject implements Serializable {
// 직렬화 가능한 객체
}
솔루션 설명
- 직렬화 가능한 객체만 직렬화하고, 객체의 직렬화 가능 여부를 확인하여 NotSerializableException을 방지할 수 있습니다.
728x90
반응형