728x90
반응형
Java 자주발생하는 오류 정리 #19
Frequent Java Cleanup Errors #19
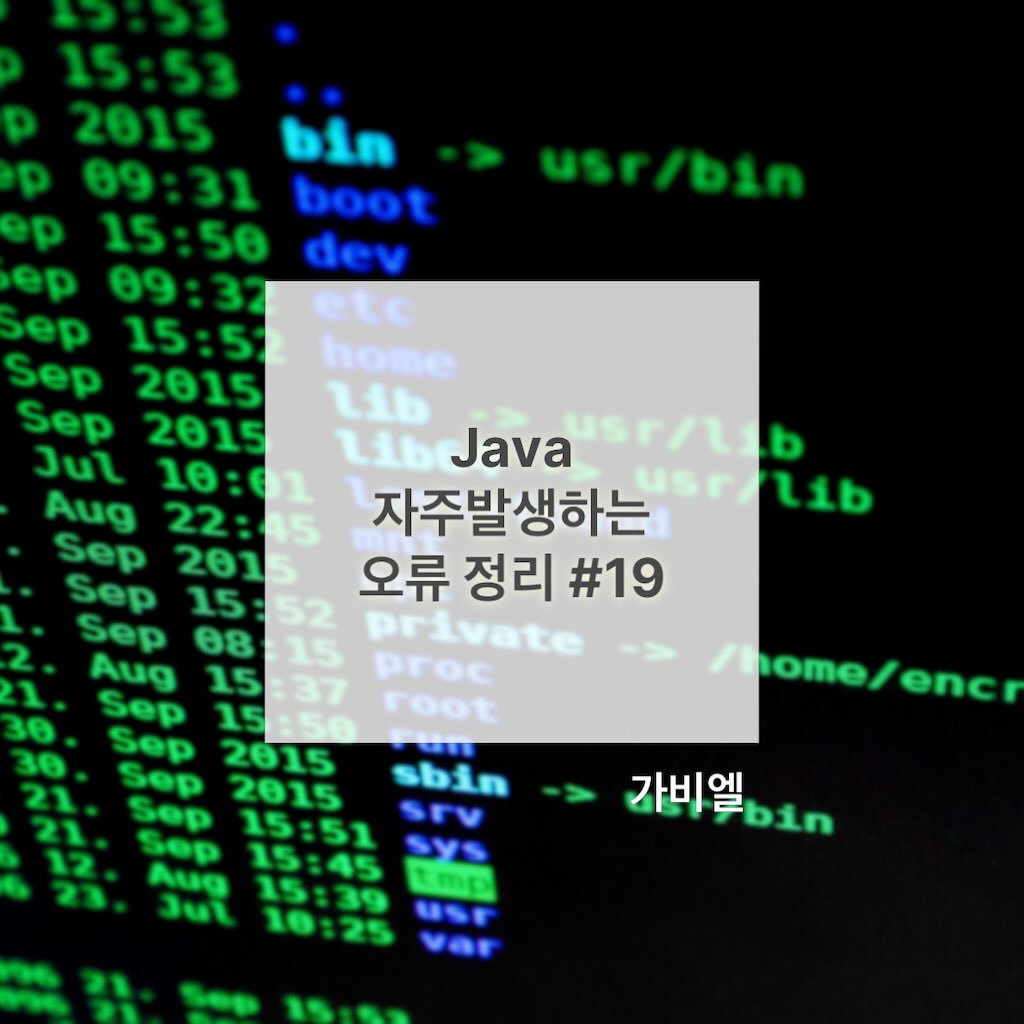
NoSuchMethodException
NoSuchAttributeException은 지정된 속성이 존재하지 않을 때 발생하는 예외입니다. 주로 속성 관리와 관련된 작업에서 발생합니다.
취약점
- NoSuchAttributeException은 주로 속성 관리와 관련이 있으며, 보안 취약점을 나타내지는 않습니다. 그러나 존재하는 속성에만 액세스하고 속성의 존재 여부를 확인하는 것이 중요합니다.
오류상황
import javax.naming.directory.*;
public class NoSuchAttributeExceptionExample {
public static void main(String[] args) {
try {
Attribute attribute = new BasicAttribute("attrName", "attrValue");
Object value = attribute.get("nonExistentAttr"); // 존재하지 않는 속성에 액세스 시도할 때 NoSuchAttributeException 발생
} catch (NoSuchAttributeException e) {
e.printStackTrace();
}
}
}
솔루션
import javax.naming.directory.*;
public class NoSuchAttributeExceptionSolution {
public static void main(String[] args) {
try {
Attribute attribute = new BasicAttribute("attrName", "attrValue");
if (attribute.get("existentAttr") != null) {
Object value = attribute.get("existentAttr"); // 존재하는 속성에만 액세스
} else {
// 존재하지 않는 속성에 대한 처리
}
} catch (NoSuchAttributeException e) {
e.printStackTrace();
}
}
}
솔루션 설명
- 존재하는 속성에만 액세스하고, 속성의 존재 여부를 확인하여 NoSuchAttributeException을 방지할 수 있습니다.
NoSuchFileException
NoSuchFileException은 파일이나 디렉토리가 존재하지 않을 때 발생하는 예외입니다. 주로 파일 시스템 작업에서 발생합니다.
취약점
- NoSuchFileException은 주로 파일 시스템 작업과 관련이 있으며, 보안 취약점을 나타내지는 않습니다. 그러나 존재하는 파일이나 디렉토리에만 액세스하고 파일의 존재 여부를 확인하는 것이 중요합니다.
오류상황
import java.nio.file.*;
public class NoSuchFileExceptionExample {
public static void main(String[] args) {
try {
Path path = Paths.get("nonexistentfile.txt");
byte[] bytes = Files.readAllBytes(path); // 존재하지 않는 파일 읽기 시도할 때 NoSuchFileException 발생
} catch (NoSuchFileException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
}
솔루션
import java.nio.file.*;
public class NoSuchFileExceptionSolution {
public static void main(String[] args) {
try {
Path path = Paths.get("existentfile.txt");
if (Files.exists(path)) {
byte[] bytes = Files.readAllBytes(path); // 존재하는 파일 읽기
} else {
// 파일이 존재하지 않을 때 처리
}
} catch (NoSuchFileException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
}
솔루션 설명
- 존재하는 파일이나 디렉토리에만 액세스하고, 파일의 존재 여부를 확인하여 NoSuchFileException을 방지할 수 있습니다.
NoSuchMethodException
NoSuchMethodException은 호출하려는 메서드가 존재하지 않을 때 발생하는 예외입니다. 주로 리플렉션 및 동적 메서드 호출에서 발생합니다.
취약점
- NoSuchMethodException은 주로 리플렉션과 관련이 있으며, 보안 취약점을 나타내지는 않습니다. 그러나 존재하는 메서드만 호출하고 메서드의 존재 여부를 확인하는 것이 중요합니다.
오류상황
import java.lang.reflect.*;
public class NoSuchMethodExceptionExample {
public static void main(String[] args) {
try {
Class<?> cls = Class.forName("java.util.ArrayList");
Method method = cls.getMethod("nonExistentMethod"); // 존재하지 않는 메서드 호출 시도할 때 NoSuchMethodException 발생
} catch (NoSuchMethodException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
솔루션
import java.lang.reflect.*;
public class NoSuchMethodExceptionSolution {
public static void main(String[] args) {
try {
Class<?> cls = Class.forName("java.util.ArrayList");
Method method = cls.getMethod("existentMethod"); // 존재하는 메서드 호출
} catch (NoSuchMethodException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
솔루션 설명
- 존재하는 메서드만 호출하고, 메서드의 존재 여부를 확인하여 NoSuchMethodException을 방지할 수 있습니다.
728x90
반응형