728x90
반응형
Java 자주발생하는 오류 정리 #16
Frequent Java Cleanup Errors #16
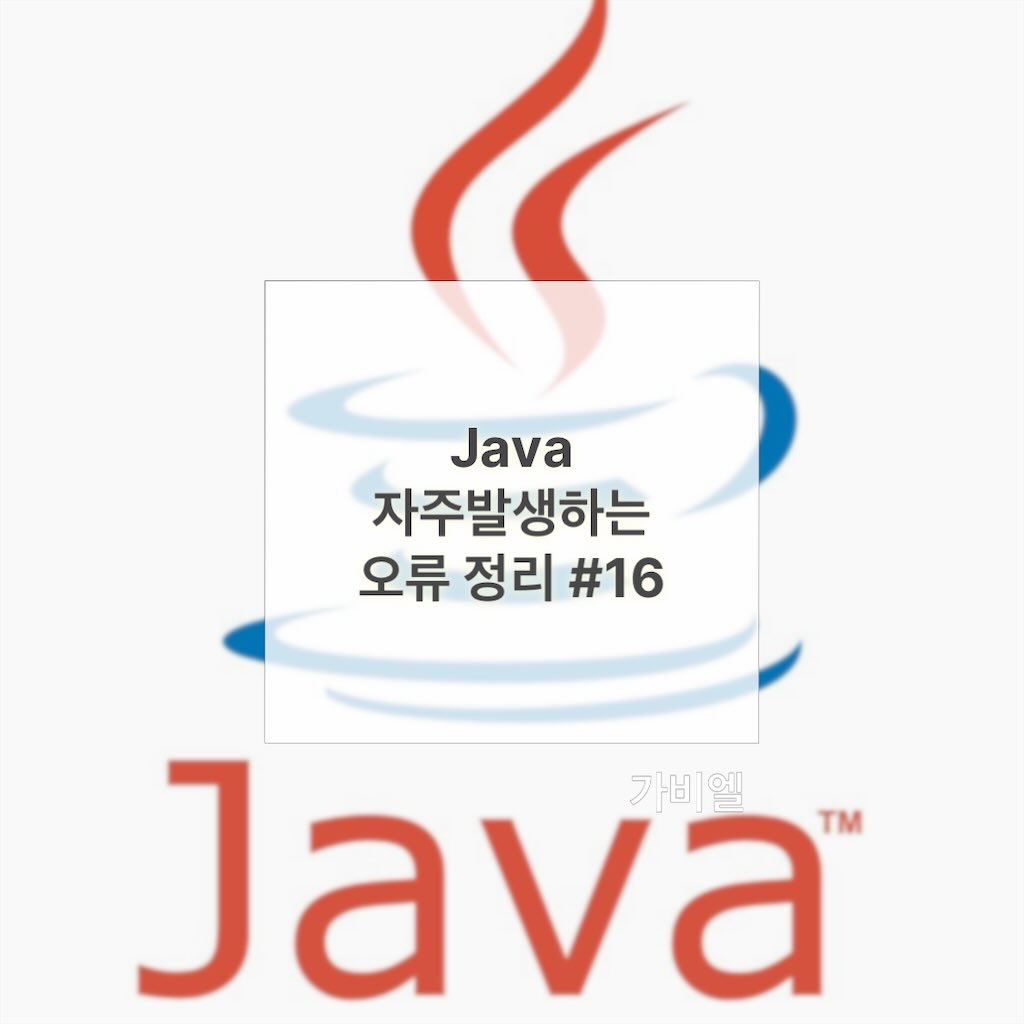
ExecutionException
ExecutionException은 작업의 실행 중에 예외가 발생했을 때 발생하는 예외입니다. 주로 병렬 실행 및 작업 관리에서 발생합니다.
취약점
- ExecutionException은 주로 작업 관리와 관련이 있으며, 보안 취약점을 나타내지는 않습니다. 그러나 작업을 실행하고 예외를 적절하게 처리하는 것이 중요합니다.
오류상황
import java.util.concurrent.*;
public class ExecutionExceptionExample {
public static void main(String[] args) {
ExecutorService executorService = Executors.newSingleThreadExecutor();
Future<String> future = executorService.submit(() -> {
// 작업 실행 중 예외 발생
throw new RuntimeException("Task execution failed");
});
try {
future.get(); // 작업 완료 대기 중 예외가 발생할 때 ExecutionException 발생
} catch (ExecutionException e) {
e.printStackTrace();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
솔루션
import java.util.concurrent.*;
public class ExecutionExceptionSolution {
public static void main(String[] args) {
ExecutorService executorService = Executors.newSingleThreadExecutor();
Future<String> future = executorService.submit(() -> {
try {
// 작업 실행
} catch (Exception e) {
// 예외 처리
throw new RuntimeException("Task execution failed");
}
});
try {
future.get(); // 작업 완료 대기 중 예외 처리
} catch (ExecutionException e) {
e.printStackTrace();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
솔루션 설명
- 작업 실행 중 발생할 수 있는 예외를 처리하고, ExecutionException을 예외 처리하여 방지할 수 있습니다.
IllegalMonitorStateException
IllegalMonitorStateException은 스레드 또는 객체의 모니터링 조건을 위반했을 때 발생하는 예외입니다. 주로 동기화 및 스레드 관리에서 발생합니다.
취약점
- IllegalMonitorStateException은 주로 스레드 관리와 관련이 있으며, 보안 취약점을 나타내지는 않습니다. 그러나 동기화 메서드를 올바르게 사용하고 모니터링 조건을 준수하는 것이 중요합니다.
오류상황
public class IllegalMonitorStateExceptionExample {
public static void main(String[] args) {
Object monitor = new Object();
try {
monitor.wait(); // wait() 메서드 호출 시 동기화되지 않은 상태에서 IllegalMonitorStateException 발생
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
솔루션
public class IllegalMonitorStateExceptionSolution {
public static void main(String[] args) {
Object monitor = new Object();
synchronized (monitor) {
try {
monitor.wait(); // 동기화된 상태에서 wait() 메서드 호출
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
솔루션 설명
- 동기화된 상태에서 wait(), notify(), notifyAll() 메서드를 사용하고, IllegalMonitorStateException을 예외 처리하여 방지할 수 있습니다.
IllegalPathStateException
IllegalPathStateException은 Path2D 클래스 또는 관련 클래스를 사용할 때 잘못된 상태일 때 발생하는 예외입니다. 주로 그래픽 및 그리기 작업에서 발생합니다.
취약점
- IllegalPathStateException은 주로 그래픽 작업과 관련이 있으며, 보안 취약점을 나타내지는 않습니다. 그러나 올바른 그래픽 상태를 유지하고 그래픽 작업을 수행하기 전에 상태를 확인하는 것이 중요합니다.
오류상황
import java.awt.geom.*;
public class IllegalPathStateExceptionExample {
public static void main(String[] args) {
Path2D path = new Path2D.Float();
path.lineTo(100, 100); // 먼저 moveTo() 메서드로 시작점을 설정하지 않고 직선 추가 시도할 때 IllegalPathStateException 발생
}
}
솔루션
import java.awt.geom.*;
public class IllegalPathStateExceptionSolution {
public static void main(String[] args) {
Path2D path = new Path2D.Float();
path.moveTo(0, 0); // 시작점 설정
path.lineTo(100, 100); // 직선 추가
}
}
솔루션 설명
- 그래픽 작업 전에 상태를 확인하고, 그래픽 상태를 유지하여 IllegalPathStateException을 방지할 수 있습니다.
728x90
반응형