728x90
반응형
Java 자주발생하는 오류 정리 #15
Frequent Java Cleanup Errors #15
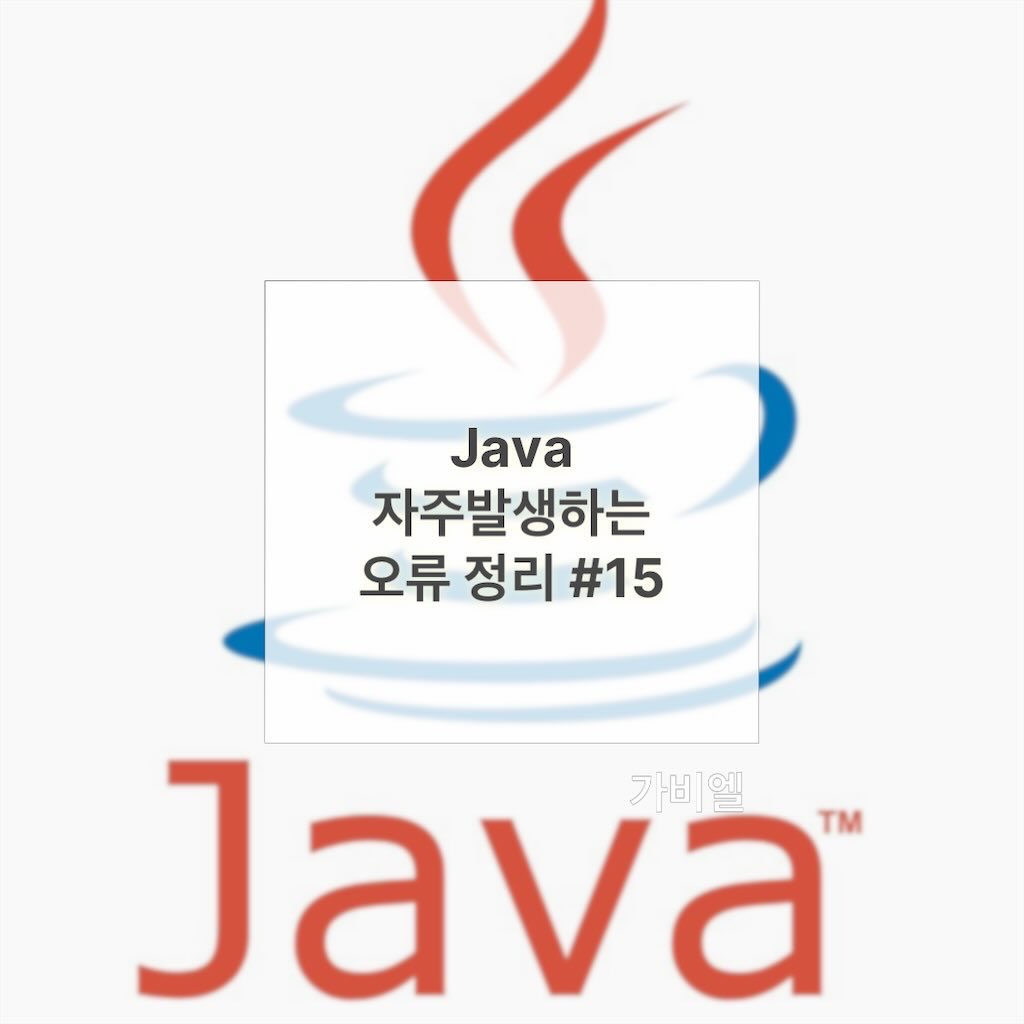
BufferUnderflowException
BufferUnderflowException은 버퍼에서 데이터를 검색하려고 할 때 버퍼에 더 이상 읽을 데이터가 없는 경우에 발생하는 예외입니다. 주로 버퍼 관련 작업에서 발생합니다.
취약점
- BufferUnderflowException은 주로 프로그래밍 오류와 관련이 있으며, 보안 취약점을 나타내지는 않습니다. 그러나 버퍼에서 데이터를 읽기 전에 읽을 데이터가 있는지 확인하는 것이 중요합니다.
오류상황
import java.nio.*;
public class BufferUnderflowExceptionExample {
public static void main(String[] args) {
ByteBuffer buffer = ByteBuffer.allocate(10);
int value = buffer.getInt(); // 더 이상 읽을 데이터가 없을 때 BufferUnderflowException 발생
}
}
솔루션
import java.nio.*;
public class BufferUnderflowExceptionSolution {
public static void main(String[] args) {
ByteBuffer buffer = ByteBuffer.allocate(10);
if (buffer.remaining() >= 4) {
int value = buffer.getInt(); // 읽을 데이터가 있는 경우에만 읽기 시도
} else {
// 읽을 데이터가 없는 경우 처리
}
}
}
솔루션 설명
- 버퍼에서 데이터를 읽기 전에 읽을 데이터가 있는지 확인하여 BufferUnderflowException을 방지할 수 있습니다.
CancellationException
CancellationException은 작업이 취소되었을 때 발생하는 예외입니다. 주로 병렬 실행 및 작업 관리에서 발생합니다.
취약점
- CancellationException은 주로 작업 관리와 관련이 있으며, 보안 취약점을 나타내지는 않습니다. 그러나 작업을 취소하고 예외를 적절하게 처리하는 것이 중요합니다.
오류상황
import java.util.concurrent.*;
public class CancellationExceptionExample {
public static void main(String[] args) {
ExecutorService executorService = Executors.newSingleThreadExecutor();
Future<String> future = executorService.submit(() -> {
while (!Thread.currentThread().isInterrupted()) {
// 작업 실행
}
throw new CancellationException();
});
future.cancel(true); // 작업 취소 시도
}
}
솔루션
import java.util.concurrent.*;
public class CancellationExceptionSolution {
public static void main(String[] args) {
ExecutorService executorService = Executors.newSingleThreadExecutor();
Future<String> future = executorService.submit(() -> {
try {
while (!Thread.currentThread().isInterrupted()) {
// 작업 실행
}
} catch (CancellationException e) {
// 작업 취소 처리
e.printStackTrace();
}
});
future.cancel(true); // 작업 취소 시도
}
}
솔루션 설명
- 작업을 취소하고, 취소 예외를 적절하게 처리하여 CancellationException을 방지할 수 있습니다.
DataFormatException
DataFormatException은 데이터의 형식이 잘못된 경우에 발생하는 예외입니다. 주로 데이터 처리 및 변환에서 발생합니다.
취약점
- DataFormatException은 주로 데이터 변환 오류와 관련이 있으며, 보안 취약점을 나타내지는 않습니다. 그러나 데이터 변환 시 올바른 형식을 사용하고 예외를 적절하게 처리하는 것이 중요합니다.
오류상황
import java.util.zip.*;
public class DataFormatExceptionExample {
public static void main(String[] args) {
byte[] data = new byte[1024];
try {
Inflater inflater = new Inflater();
inflater.setInput(data);
byte[] buffer = new byte[1024];
int bytesRead = inflater.inflate(buffer); // 잘못된 형식의 데이터 변환 시도
} catch (DataFormatException e) {
e.printStackTrace();
}
}
}
솔루션
import java.util.zip.*;
public class DataFormatExceptionSolution {
public static void main(String[] args) {
byte[] data = new byte[1024];
try {
Inflater inflater = new Inflater();
inflater.setInput(data);
byte[] buffer = new byte[1024];
try {
int bytesRead = inflater.inflate(buffer);
// 데이터 변환 작업
} catch (DataFormatException e) {
// 잘못된 형식의 데이터 변환 처리
e.printStackTrace();
}
} catch (DataFormatException e) {
e.printStackTrace();
}
}
}
솔루션 설명
- 데이터 변환 작업을 수행하기 전에 데이터의 형식을 확인하고, DataFormatException을 예외 처리하여 방지할 수 있습니다.
728x90
반응형